Published on: | By NI18 Team
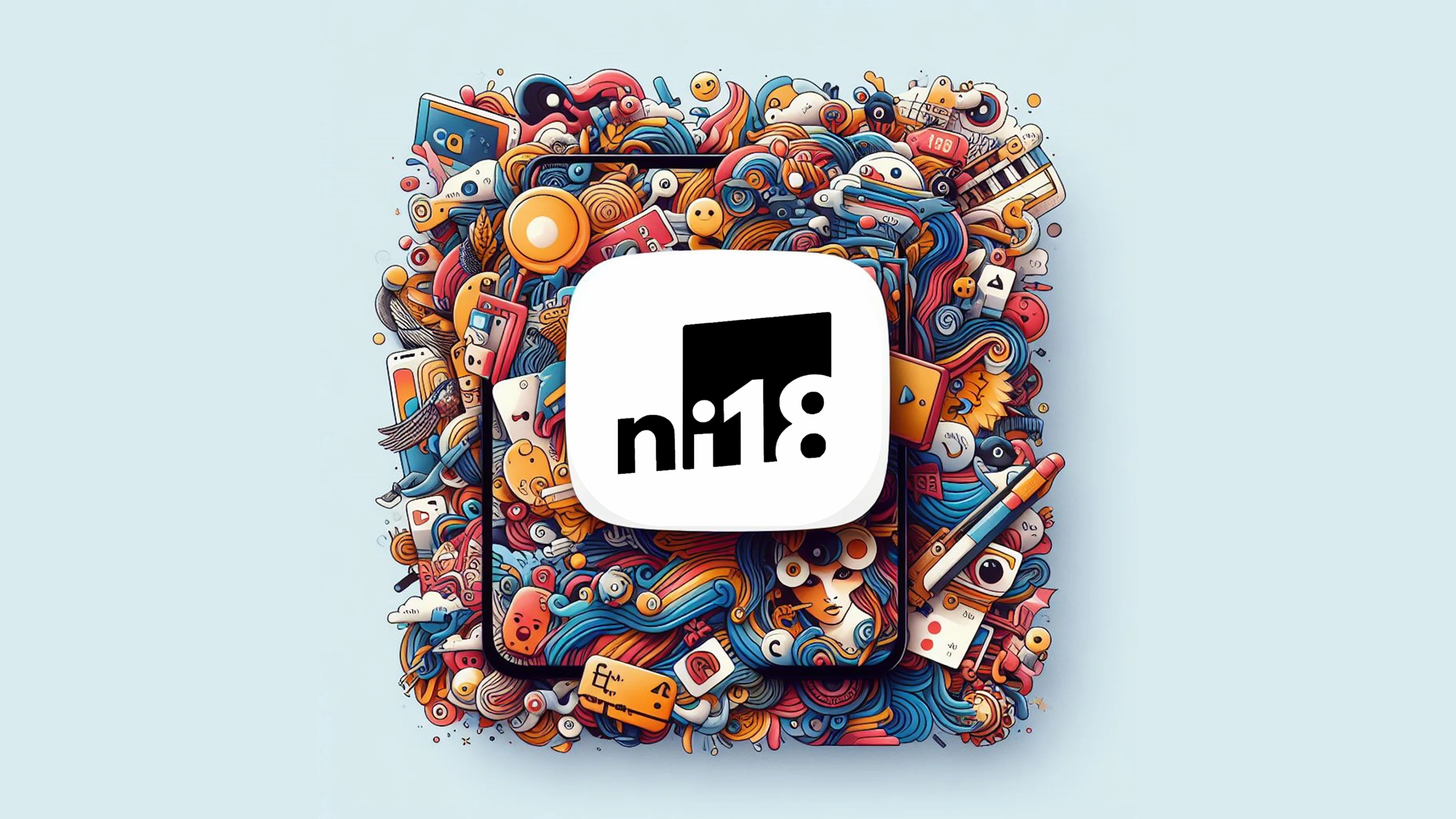
Introduction
JavaScript is one of the most popular programming languages in the world. Whether you are a beginner or a seasoned developer looking to expand your skills, this roadmap will help you navigate the world of JavaScript—from the basics to advanced concepts. In this guide, we will break down each step and provide practical tips and resources to help you learn effectively.
JavaScript is not just a language for web browsers; it is also used on the server side (Node.js), in mobile app development (React Native, Ionic), and even for building desktop applications (Electron). Its versatility has made it a must-learn skill for anyone interested in modern software development.
In this guide, we will walk you through a clear, step-by-step path to master JavaScript. Whether you want to build interactive web pages or develop complex applications, this roadmap will provide you with the knowledge and skills required to succeed.
Why Learn JavaScript?
JavaScript is everywhere in today’s digital world. Here are a few compelling reasons why you should consider learning it:
- High Demand: Tech companies worldwide consistently seek JavaScript skills for front-end, back-end, and full-stack development roles.
- Versatility: Use JavaScript for a vast range of applications: dynamic web development, mobile app creation, server-side programming, game development, and more.
- Rich Ecosystem & Community: A massive and active global community means an abundance of tutorials, libraries, frameworks, documentation, and readily available support.
- Career Opportunities: JavaScript developers often find a wide range of job opportunities with competitive salaries and growth potential.
- Core Web Technology: Alongside HTML and CSS, JavaScript is one of the three core technologies of the World Wide Web.
Getting Started with JavaScript Basics
Before you dive into more complex topics, you need to build a strong foundation in the basics. Here’s how to get started:
Essential Concepts
-
Variables and Data Types:
- Understand how to declare variables using
var
,let
, andconst
, and their differences (scope, hoisting). - Learn about JavaScript's primitive data types: numbers, strings, booleans,
null
,undefined
,symbol
, andbigint
. - Explore complex data types: objects and arrays.
- Understand how to declare variables using
-
Operators:
- Get familiar with arithmetic (
+
,-
,*
,/
,%
), assignment (=
,+=
), comparison (==
,===
,!=
,!==
,>
,<
), logical (&&
,||
,!
), and ternary (? :
) operators.
- Get familiar with arithmetic (
-
Control Structures:
- Learn how to use conditional statements (
if
,else if
,else
,switch
) to make decisions in your code. - Master loops (
for
,while
,do...while
,for...in
,for...of
) for repetitive tasks.
- Learn how to use conditional statements (
-
Functions:
- Understand how to define and call functions (function declarations, function expressions, arrow functions).
- Explore the concepts of parameters, arguments, return values, and function scope.
-
Basic DOM Manipulation:
- Learn how to select HTML elements using methods like
getElementById
,getElementsByClassName
,querySelector
, etc. - Understand how to modify element content (
innerHTML
,textContent
), attributes, and styles. - Learn about events (e.g., clicks, key presses, mouse movements) and how to attach event listeners to respond to user actions.
- Learn how to select HTML elements using methods like
Hands-On Practice
- Write Simple Scripts: Start by writing small scripts that perform basic tasks, like changing text on a webpage or performing simple calculations.
- Use Online Editors & Browser Console: Platforms like CodePen, JSFiddle, or your browser's developer console are great places to experiment and practice.
- Build Small Projects: Create simple projects like a basic calculator, a to-do list application, or an interactive quiz to solidify your learning and see tangible results.
Diving Deeper: Intermediate Concepts
Once you have a solid grasp of the basics, it’s time to explore intermediate topics that will help you write more efficient, organized, and scalable code.
Core JavaScript Concepts
-
Objects and Arrays In-Depth:
- Learn advanced techniques for creating and manipulating objects (constructors, prototypes,
this
keyword). - Master array methods (
map
,filter
,reduce
,forEach
,find
,sort
, etc.) for powerful data manipulation.
- Learn advanced techniques for creating and manipulating objects (constructors, prototypes,
-
Functions as First-Class Citizens:
- Explore the concept of higher-order functions—functions that operate on other functions, either by taking them as arguments or by returning them.
- Understand callbacks and their role in asynchronous operations and event handling.
-
Scope and Closures:
- Delve deeper into how JavaScript handles variable scope (global, function/local, block scope with
let
/const
) and hoisting. - Learn about closures, a powerful feature where an inner function has access to the outer (enclosing) function’s variables, even after the outer function has finished executing.
- Delve deeper into how JavaScript handles variable scope (global, function/local, block scope with
-
Error Handling:
- Understand how to effectively handle errors and exceptions using
try...catch...finally
blocks. - Learn about different types of errors and the importance of writing robust code that can gracefully handle unexpected situations.
- Understand how to effectively handle errors and exceptions using
-
The `this` Keyword:
- Understand the different ways the
this
keyword behaves depending on how a function is called (global context, as a method, as a constructor, withcall
/apply
/bind
, in arrow functions).
- Understand the different ways the
Working with the DOM More Effectively
-
Advanced DOM Manipulation:
- Practice creating, inserting, removing, and replacing HTML elements dynamically.
- Learn about traversing the DOM tree (parent, child, sibling nodes).
-
Event Delegation and Management:
- Explore event bubbling and capturing.
- Understand event delegation for more efficient handling of events on multiple elements.
-
Building Interactive Interfaces:
- Combine your knowledge of the DOM and events to create more complex interactive web pages and components.
- Build projects like dynamic forms with validation, interactive image galleries, or simple single-page application (SPA) features.
Mastering Advanced JavaScript
After mastering the intermediate topics, you are ready to move on to advanced JavaScript. This stage focuses on modern JavaScript features (ES6 and beyond), design patterns, and best practices for writing professional-grade code.
ES6+ Features (Modern JavaScript)
- Arrow Functions: Deepen your understanding of their syntax, lexical
this
binding, and use cases. - Template Literals: Master multi-line strings and embedded expressions for cleaner string manipulation.
- Destructuring Assignment: Efficiently extract values from arrays or properties from objects into distinct variables.
- Spread and Rest Operators (
...
): Understand their use for expanding iterables, merging objects/arrays, and handling variable numbers of function arguments. - Default Parameters: Define default values for function parameters.
- Classes: Learn the syntactical sugar for creating constructor functions and working with prototypes in an object-oriented style.
- Modules (Import/Export): Master ES6 modules to organize your code into reusable and maintainable pieces.
- Promises & Async/Await (Asynchronous Programming):
- Thoroughly understand Promises for managing asynchronous operations and avoiding "callback hell."
- Learn how
async/await
provides a cleaner, more synchronous-looking syntax for working with Promises.
- Iterators and Generators: Understand how to create custom iteration behaviors.
- Map, Set, WeakMap, WeakSet: Learn about these useful data structures.
Asynchronous Programming Deep Dive
- Event Loop, Call Stack, Callback Queue: Understand the underlying mechanism of how JavaScript handles asynchronous operations.
- Advanced Promise Patterns: Learn about
Promise.all()
,Promise.race()
,Promise.allSettled()
,Promise.any()
. - Fetch API: Master making HTTP requests to APIs using the modern Fetch API.
Modern JavaScript Tools & Practices
- Package Managers (npm/yarn): Become proficient in using npm or yarn to manage project dependencies and scripts.
- Bundlers and Build Tools (Webpack, Parcel, Rollup): Learn how these tools bundle your JavaScript modules, CSS, and other assets for efficient delivery to the browser. Understand concepts like code splitting and tree shaking.
- Linters and Formatters (ESLint, Prettier): Use these tools to enforce code style consistency and catch potential errors early.
- Testing Frameworks (Jest, Mocha, Cypress): Explore and practice writing unit, integration, and end-to-end tests for your JavaScript code to ensure quality and reliability.
- Transpilers (Babel): Understand how tools like Babel allow you to write modern JavaScript (ES6+) and transpile it down to older versions (like ES5) for broader browser compatibility.
- Version Control (Git): Essential for any developer. Master Git for tracking changes, collaboration, and managing code history.
- Browser Developer Tools: Become an expert at using your browser's dev tools for debugging, performance profiling, and inspecting the DOM.
Exploring JavaScript Frameworks and Libraries
After gaining a strong command of core JavaScript and modern development practices, it’s beneficial to explore frameworks and libraries. These tools can significantly accelerate development, provide structure, and help you build complex, scalable applications more efficiently.
Popular Front-End Frameworks/Libraries
- React:
- A declarative, efficient, and flexible JavaScript library for building user interfaces, developed by Facebook.
- Focuses on creating reusable UI components and managing application state (often with tools like Redux or Zustand).
- Angular:
- A comprehensive, opinionated framework developed and maintained by Google, built with TypeScript.
- Ideal for building large-scale, complex enterprise applications, offering a complete solution for routing, state management, and more.
- Vue.js:
- A progressive and approachable framework known for its simplicity, flexibility, and excellent documentation.
- Easy to integrate into existing projects or use to build full-fledged SPAs.
- Svelte:
- A newer approach where Svelte compiles your code to highly efficient imperative code that directly manipulates the DOM, rather than using a virtual DOM.
Server-Side JavaScript (Node.js)
- Node.js:
- A JavaScript runtime built on Chrome's V8 engine that allows you to run JavaScript on the server side.
- Enables building scalable network applications, REST APIs, command-line tools, and more.
- Express.js:
- A minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It's the de facto standard for many Node.js projects.
- Other Node.js Frameworks: Explore others like NestJS (for building efficient, scalable server-side applications with TypeScript), Koa, or Fastify.
Other Useful Tools and Libraries
- State Management Libraries: Redux, Zustand, Pinia (for Vue), NgRx (for Angular) – for managing complex application state.
- Data Fetching/GraphQL Clients: Apollo Client, React Query, SWR – for efficient data fetching and caching.
- Utility Libraries: Lodash, Moment.js/Day.js – for common utility functions and date/time manipulation.
- TypeScript: While not a framework, it's a superset of JavaScript that adds static types. Highly recommended for larger projects to improve code quality, maintainability, and developer experience.
Putting It All Together & Continuous Learning
As you progress through this roadmap, remember that consistent practice is key. Theoretical knowledge is important, but applying what you learn by building projects is what truly solidifies your skills.
- Build Diverse Projects: Start with small, focused projects for each concept. Gradually work on more complex applications that integrate multiple concepts and technologies. Examples: a weather app, a movie database browser, a real-time chat application, an e-commerce site.
- Contribute and Collaborate: Join coding communities (like GitHub, Dev.to, Stack Overflow). Participate in hackathons or contribute to open-source projects. Collaborating with others is a great way to learn and improve.
- Stay Updated with Trends: JavaScript and its ecosystem are constantly evolving. Follow influential developers and organizations, subscribe to newsletters (e.g., JavaScript Weekly), read blogs, and participate in webinars or conferences to stay updated on the latest features, tools, and best practices.
- Review and Refactor Your Code: Regularly revisit your old code. You'll often find ways to improve it based on new knowledge. Refactoring helps in understanding design patterns and writing cleaner, more efficient code.
- Seek Feedback: Don't be afraid to share your code and ask for feedback from more experienced developers. Code reviews are invaluable for growth.
Additional Resources and Next Steps
To further your journey in learning JavaScript, consider these valuable resources:
- Online Courses & Interactive Platforms:
- Platforms like Udemy, Coursera, Pluralsight, freeCodeCamp, The Odin Project, Scrimba, and Codecademy offer excellent structured JavaScript courses for all levels.
- Official Documentation and Reference:
- The Mozilla Developer Network (MDN) Web Docs is an indispensable and comprehensive resource for JavaScript and web technologies.
- ECMAScript specification (for the very brave and curious).
- Influential Books:
- “Eloquent JavaScript” by Marijn Haverbeke (available online for free).
- “You Don’t Know JS Yet” (2nd Edition) series by Kyle Simpson (challenges deep understanding).
- "JavaScript: The Good Parts" by Douglas Crockford (classic, though a bit dated, still insightful).
- Community and Forums:
- Join communities like Stack Overflow (for specific questions), Reddit’s r/javascript and r/learnjavascript, Dev.to, Hashnode, or local coding meetups to ask questions, share experiences, and collaborate with fellow developers.
Learning JavaScript is a rewarding journey that can open many doors in the vast world of software development. This roadmap provides a structured path from the fundamentals to advanced topics, covering essential knowledge to become proficient in JavaScript. Remember that the key to mastering any programming language is consistent practice, building projects, and continuous learning.
Start with the fundamentals, build your skills with intermediate topics, and then dive into the advanced features and ecosystem that modern JavaScript offers. Once you have a solid grasp of the language, explore frameworks and libraries to build powerful, scalable applications.
Whether you are building interactive web pages, developing mobile apps, or creating server-side applications, JavaScript is an essential tool in your developer toolkit. Use this roadmap as your guide, be patient with yourself, and enjoy your journey toward becoming a skilled JavaScript developer!