Published on: | By Nitin | Last Updated:
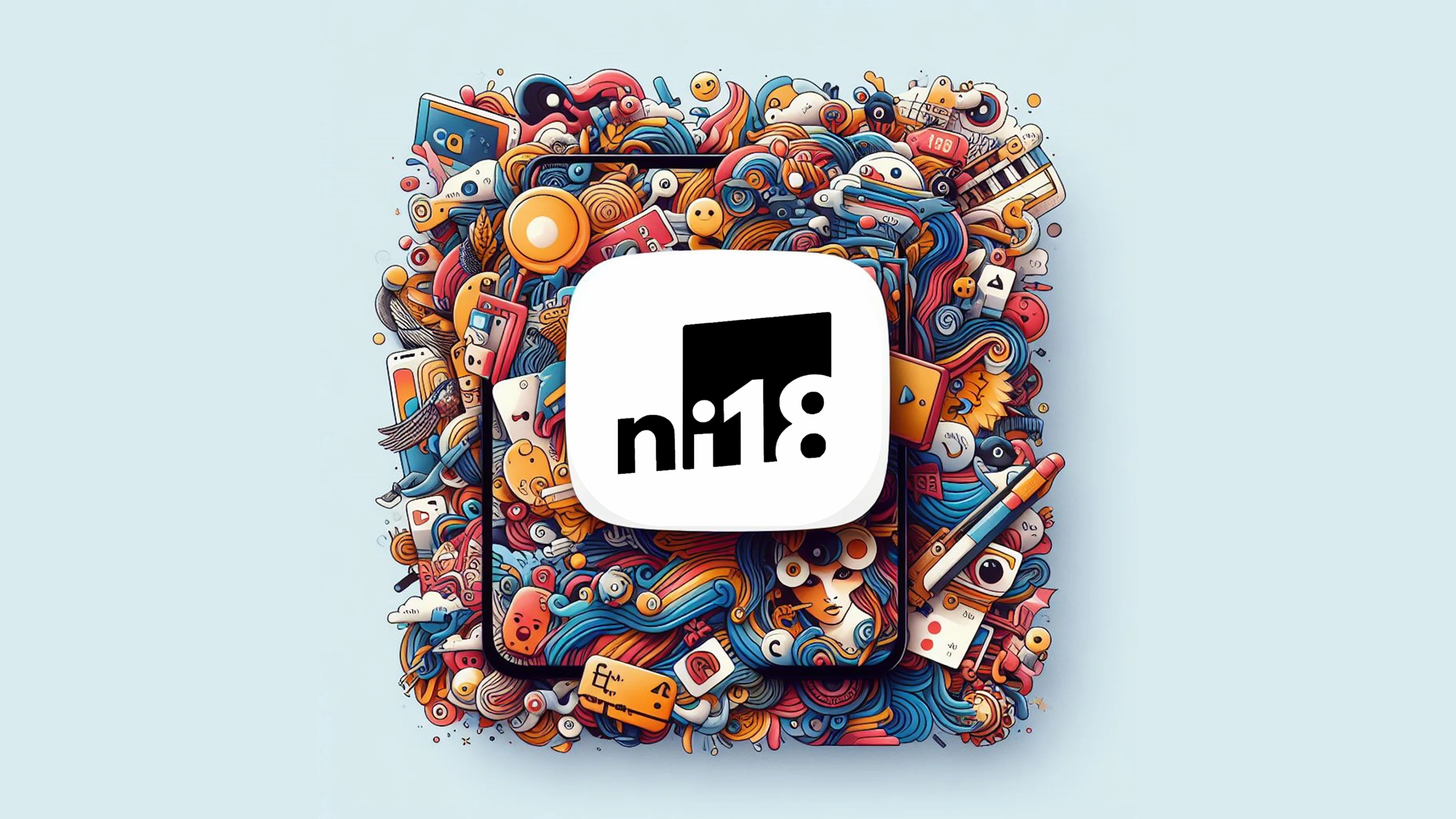
When it comes to web development, JavaScript has been a dominant language for decades. Recently, however, TypeScript, a superset of JavaScript, has gained significant traction among developers. Both languages have their unique strengths and use cases. This article will explore the key differences between TypeScript and JavaScript, focusing on performance, scalability, error checking, community support, and their suitability for various projects.
What is JavaScript?
JavaScript is a versatile, dynamic programming language primarily used to create interactive web applications. It is supported by all modern web browsers and is an essential part of the web development trifecta (HTML, CSS, JavaScript). Known for its flexibility, JavaScript enables developers to build everything from simple websites to complex applications. Its event-driven, non-blocking nature makes it efficient for user interface interactions and server-side operations with Node.js.
What is TypeScript?
TypeScript is a statically typed superset of JavaScript developed and maintained by Microsoft. It builds upon JavaScript by adding optional static typing, interfaces, enums, generics, and other advanced tooling capabilities. TypeScript code compiles down to plain JavaScript, making it compatible with all environments where JavaScript runs (browsers, Node.js, etc.). It is particularly beneficial for large-scale projects requiring maintainable, robust, and scalable codebases, as it helps catch errors during development rather than at runtime.
Performance Comparison
In terms of raw runtime performance, there is no significant inherent difference between TypeScript and JavaScript. This is because TypeScript is a compiled language, meaning it must first be converted (transpiled) into standard JavaScript before it can be executed by a browser or a Node.js environment. Since the final executed code is JavaScript, the performance during runtime depends entirely on the quality and optimization of that resulting JavaScript code, as well as the efficiency of the JavaScript engine itself.
Key Takeaways:
- TypeScript compiles into JavaScript, so its runtime performance is identical to that of well-written JavaScript.
- Any perceived performance differences usually stem from the development process (e.g., type checking overhead during compilation for TypeScript) or how developers leverage TypeScript's features to write more optimized or less error-prone JavaScript.
- The efficiency of your code depends more on algorithmic choices, optimization techniques, and best practices rather than the choice between TypeScript and JavaScript for runtime speed.
Scalability and Maintainability
Scalability and maintainability are areas where TypeScript often demonstrates significant advantages, especially as projects grow in size and complexity.
Benefits of TypeScript:
- Static Typing: This is TypeScript's flagship feature. By defining types for variables, function parameters, and return values, developers can catch a large class of errors at compile time (during development) rather than at runtime. This leads to more robust code.
- Improved Readability and Self-Documentation: Type annotations make the code more self-documenting. It's easier for developers (including future you or new team members) to understand the data structures and expected inputs/outputs of functions.
- Better Tooling and IDE Support: TypeScript offers enhanced support for Integrated Development Environments (IDEs) like VS Code. This includes features like intelligent autocompletion, precise refactoring capabilities, and real-time error detection, which significantly boost developer productivity.
- Easier Refactoring: Static types make refactoring large codebases safer and more manageable, as the compiler can help identify parts of the code affected by changes.
- Interfaces and Generics: These features allow for the creation of reusable and flexible code components, promoting better software design.
JavaScript, with its dynamic typing, offers flexibility that can be beneficial for rapid prototyping and smaller projects. However, this flexibility can become a challenge to maintain as projects grow. The lack of static type checking may lead to runtime errors that are harder to track down and debug, potentially increasing development time and costs in the long run.
Use Case Comparison:
- TypeScript: Ideal for large-scale, complex applications, projects with multiple developers, and situations where long-term maintainability and code quality are paramount.
- JavaScript: Suitable for smaller projects, scripts, rapid prototyping, and when the development team is small and highly experienced with JavaScript's nuances.
Error Checking and Debugging
One of TypeScript’s most significant advantages is its robust error-checking capability, primarily through static type checking. This proactive approach helps developers identify and fix many common issues during the development phase, before the code is even run.
Example:
JavaScript Code (Potential Runtime Error):
function addNumbers(num1, num2) {
return num1 + num2; // If num2 is a string, this causes concatenation
}
console.log(addNumbers(5, "3")); // Output: "53" - likely not the intended behavior
TypeScript Code (Compile-Time Error):
function addNumbers(num1: number, num2: number): number {
return num1 + num2;
}
// console.log(addNumbers(5, "3"));
// This would cause a compile-time error:
// Argument of type 'string' is not assignable to parameter of type 'number'.
console.log(addNumbers(5, 3)); // Output: 8
In the JavaScript example, the lack of type enforcement can lead to unexpected behavior (like string concatenation instead of addition) that might only be discovered during testing or in production. TypeScript’s static typing prevents such issues by flagging incompatible data types during the compilation step, prompting the developer to fix them immediately.
Benefits of TypeScript for Error Checking:
- Detects type-related errors and many other common bugs before runtime.
- Significantly reduces the time spent on debugging runtime issues.
- Improves overall code quality and reliability.
- Provides clearer error messages that pinpoint the source of the problem.
Community and Ecosystem
Both TypeScript and JavaScript boast vibrant, extensive communities and rich ecosystems of libraries, frameworks, and tools.
JavaScript, being the older and foundational language of the web, naturally has a larger historical footprint. This translates to an incredibly broad range of libraries, frameworks (like jQuery, though less common now), and resources developed over many years. Almost any problem a web developer might face has likely been addressed by a JavaScript library.
TypeScript’s popularity has grown rapidly, especially in the professional development sphere and for larger applications. Its ecosystem is also robust and continuously expanding. Most popular JavaScript libraries and frameworks now have excellent TypeScript support, either natively or through community-maintained type definition files (from DefinitelyTyped/@types).
Why Developers Choose TypeScript:
- Modern Language Features: Built-in support for interfaces, enums, generics, decorators, and other features simplify complex software development and promote better design patterns.
- Seamless Integration: Works exceptionally well with popular modern frameworks like React, Angular (which is written in TypeScript), and Vue.js.
- Strong Community Support: A rapidly growing and active community ensures continuous improvements, robust tooling, and readily available help.
- Improved Developer Experience (DX): The combination of static typing and excellent IDE support often leads to a more productive and less frustrating development experience.
Why JavaScript Remains Popular:
- Simplicity for Small Tasks: No compilation step is required; code runs directly in the browser or Node.js, making it quick for scripting and small projects.
- Flexibility: Dynamic typing can be advantageous for rapid prototyping and situations where strict type definitions might feel cumbersome initially.
- Vast Legacy and Resources: An enormous amount of existing JavaScript code, tutorials, and libraries are available.
- Lower Barrier to Entry for Beginners: Often perceived as easier to pick up initially due to the lack of a compilation step and type system.
Real-World Applications
TypeScript:
TypeScript is widely adopted in large-scale applications where maintainability, code quality, and collaboration among large teams are critical. Its strong typing system helps manage complexity and reduce bugs in substantial codebases. Companies like Microsoft (its creator), Google (for Angular), Airbnb, Asana, and Slack rely on TypeScript for its static typing and tooling benefits. Its compatibility with modern frameworks makes it a top choice for developing scalable single-page applications (SPAs), server-side applications with Node.js (often using frameworks like NestJS), and even desktop applications (with Electron).
JavaScript:
JavaScript remains an excellent choice for a wide array of projects, particularly small to medium-sized applications, quick scripts, and situations where rapid development and simplicity are priorities. It is ideal for:
- Prototyping and quick iterations.
- Adding interactivity to websites.
- Server-side scripting with Node.js for simpler APIs or microservices.
- Scenarios where the development team is small or prefers the flexibility of dynamic typing.
- Many existing legacy systems and a vast number of open-source libraries are written in JavaScript.
Choosing Between TypeScript and JavaScript
The decision between TypeScript and JavaScript ultimately depends on your project’s specific requirements, the size and expertise of your team, and your long-term goals. Below are some guidelines to help you make an informed choice:
Choose JavaScript If:
- You are building small-scale applications, simple websites, or scripts.
- Rapid prototyping and quick development cycles are the highest priority.
- Your team is small and highly proficient in JavaScript, comfortable with dynamic typing.
- A build/compilation step is undesirable or adds too much overhead for the project's scope.
- You are working on a legacy project where introducing TypeScript might be too disruptive.
Choose TypeScript If:
- You are developing large, complex, or enterprise-level applications.
- You want to catch errors early in the development process and reduce runtime bugs.
- Maintainability, scalability, and code quality are top priorities for the long term.
- You are working in a team environment, especially with developers of varying experience levels, as types improve collaboration and code understanding.
- You prefer the enhanced tooling, autocompletion, and refactoring capabilities offered by statically typed languages.
- You are already using a framework like Angular, which is built with TypeScript, or want to leverage TypeScript's benefits with React or Vue.js.
Both TypeScript and JavaScript are powerful and valuable tools for web and application development. While JavaScript’s simplicity and flexibility make it a great choice for certain projects, TypeScript’s static typing and advanced features provide significant advantages for building robust, large-scale, and collaborative applications.
Ultimately, the best choice depends on a careful evaluation of your project’s complexity, long-term goals, and team preferences. Many projects also successfully adopt TypeScript incrementally. Regardless of the language, focusing on writing clean, efficient, and maintainable code, along with following best practices, will set you up for success in your development journey.
Read More About TypeScript vs JavaScript (Performance Focus) or Learning React in 2025.